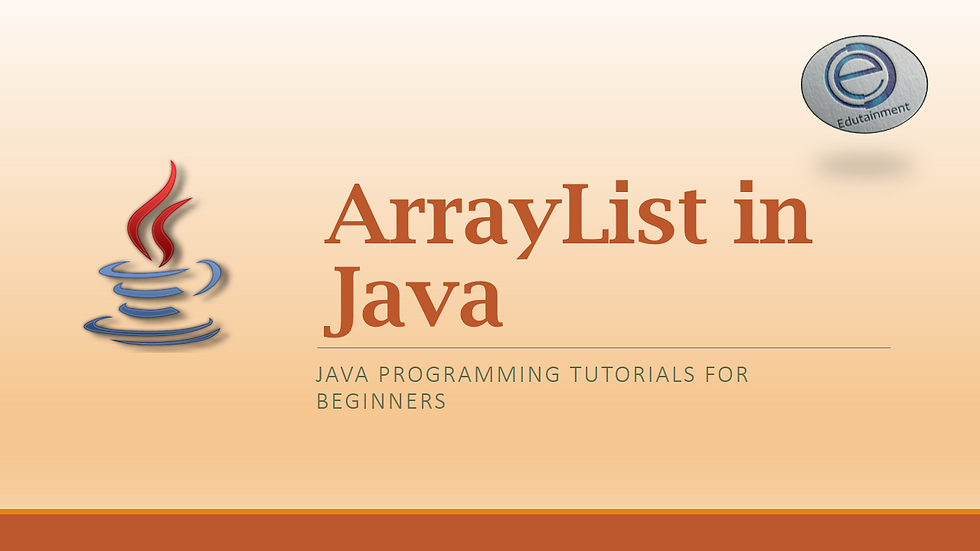
Arrays are widely used in java but they have some disadvantages for example the fixed size of array, means array size cannot be modified once declared. Similarly addition and deletion of array elements are also very difficult task.
To resolve the issue Java introduced collections.
ArrayList is a collection. A collection is a single object that groups multiple elements into single unit. Collection are used to store, retrieve and manipulate aggregate data.
Java provides a set of collection interfaces to create different type of collections.
Characteristics
The ArrayList class is a frequently used collection that has the following characteristics.
1. It is flexible in used. It size can be increase and decrease during the program.
2. It provide many useful method to manipulate the data as per need.
3. Modification (insertion and deletion) of data is simpler.
4. It can be traversed by loops.
Create an ArrayList and Add Values to it.
import java.util.ArrayList;
/**
*
* @author Haseeb Hussain
*/
public class CreatArrayList {
public static void main( String arg[]){
ArrayList numbers = new ArrayList();
numbers.add(6);
numbers.add(4);
numbers.add(5);
//add value to specific index
numbers.add(1,7);
System.out.println(numbers);
}
}
Output:
[6, 4, 5]
Add values of one collection to another
import java.util.ArrayList;
/**
*
* @author Haseeb Hussain
*/
public class CreatArrayList {
public static void main( String arg[]){
ArrayList numbers = new ArrayList();
numbers.add(6);
numbers.add(4);
numbers.add(5);
// second arraylist
ArrayList numbers2 = new ArrayList();
numbers2.add(3);
numbers2.add(9);
numbers.add(2);
// add values of numbers2 to numbers
numbers.addAll(numbers2);
System.out.println(numbers);
}
}
Komentar