Authentication Using Shared Preference | Part 1 | Android Tutorial 2020
- Haseeb Hussain
- Jun 28, 2020
- 2 min read
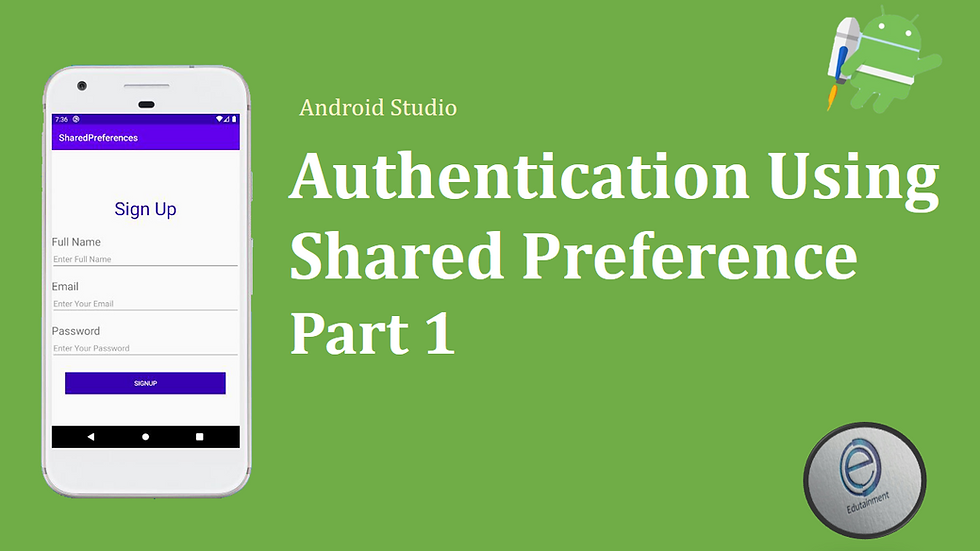
Shared Preference is a local storage (file) used to store small amount of data. It's object point to a file which contains data in key-value pair and it provides simple methods to read and write them into that file instance.
Type of Shared Preference
1. getSharedPreferences() - using this method you can create multiple preferences where each preference identified by name and can be call from any context in your app.
2. getPreferences() - you can use this if you want only one shared preference for a single activity. This preference only associated to the same activity and there is no need to specify a name.
In this example we will create a simple authentication system which includes
Signup User.
Login User
Logout User
Check if your logged in or not.
1 - Create a new android project.
2 - For Signup
create a new activity for signup activity and name it Signup.
Now copy paste the code of activity_signup.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".Signup"
android:padding="18dp"
>
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="100dp"
android:layout_marginBottom="30dp"
android:gravity="center"
android:text="Sign Up"
android:textColor="@color/colorPrimaryDark"
android:textSize="40sp"
android:textStyle="bold"
/>
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Full Name"
android:textSize="24sp" />
<EditText
android:id="@+id/fullname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPersonName"
android:hint="Enter Full Name"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Email"
android:textSize="24sp"
android:layout_marginTop="20dp"/>
<EditText
android:id="@+id/email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textEmailAddress"
android:hint="Enter Your Email" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Password"
android:textSize="24sp" />
<EditText
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword"
android:hint="Enter Your Password" />
<Button
android:id="@+id/button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Signup"
android:layout_margin="30dp"
android:background="@color/colorPrimaryDark"
android:textColor="#fff"
android:onClick="signup"
/>
</LinearLayout>
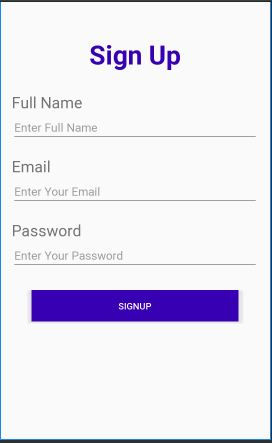
Now copy the code in Signup.java file.
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class Signup extends AppCompatActivity {
EditText mName,mEmail,mPassword;
SharedPreferences ref;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_signup);
mName = findViewById(R.id.fullname);
mEmail = findViewById(R.id.email);
mPassword = findViewById(R.id.password);
// initialize the shared preference in private mode
ref = getSharedPreferences("myapp",MODE_PRIVATE);
}
public void signup(View view) {
//getting edit text values
String name = mName.getText().toString();
String email = mEmail.getText().toString();
String password = mPassword.getText().toString();
//save the values to shared preferences
ref.edit().putString("name",name).apply();
ref.edit().putString("email",email).apply();
ref.edit().putString("password",password).apply();
ref.edit().putBoolean("login",true).apply();
//call the main activity after signup successfull
Intent i = new Intent(Signup.this,MainActivity.class);
startActivity(i);
}
}
after signup the Main Activity will be open.
- Setup the main activity. To do so copy paste the code in activity_main.xml file
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Welcome"
android:textColor="#000"
android:textSize="40dp"
android:textStyle="bold"
android:gravity="center"
android:layout_gravity="center"
android:layout_marginTop="30dp"
/>
<TextView
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:textSize="30dp"
android:text="Name"
android:textStyle="bold"
/>
</LinearLayout>
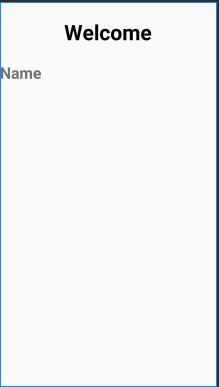
this view have two TextViews one to show the welcome text and another will be used to show the logged in user name.
After that go to MainActivity.java file and paste the code there
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
TextView mName;
SharedPreferences ref;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mName = findViewById(R.id.name);
// initialize the shared preference
ref = getSharedPreferences("myapp",MODE_PRIVATE);
// set the name to edit text after getting it from preferences
mName.setText(ref.getString("name",""));
}
}
in this activity we get the signed up user name from shared preferences and set the name to TextView.
Run the application to test it.
Here we complete the signup method. This is part 1 will be continue to create login and logout in next tutorial.
Comments